Parallax Google Analytics tracking
This posts describes how to use Google Analytics to track where a user scrolls to when using a parallax effect.
Google Analytics Event tracking
Google provides an Event Tracking API which can be used to track custom events. We will use it to track where a user scrolls to on a Parallax based website.
Scenario
Website:
- We have a single page website
- The Google Analytics Javascript is included in the page
- We have a navigation menu with the links Home, Blog and Contact
- Clicking a link will scroll the page to the correct section
We will track:
- which menu item has been clicked in Google Analytics
- which section a user manually scrolls to in Google Analytics
HTML
Each section of the page in the parallox effect will be placed in a div
with a unique id:
<div id="home">...</div>
<div id="blog">...</div>
<div id="contact">...</div>
The menu uses a typical ul
unorder list:
<ul>
<li class="home"><a href="#home">Home</a></li>
<li class="blog"><a href="#blog">Blog</a></li>
<li class="contact"><a href="#contact">contact</a></li>
</ul>
Tracking scroll to section
Waypoints javascript library will be used, make sure it is installed.
It is essential that each parallax section has a unique id
attribute set as in the sample html.
Add the below script to the bottom of the page.
<script>
/* Used to track sent events to stop duplicates */
var parallaxEventLog = {};
/* Home section view tracking */
new Waypoint({
element: document.getElementById('home'),
handler: function() {
if(!parallaxEventLog['home']) {
ga('send', 'event', 'homepage', 'section_view', 'home');
parallaxEventLog['home'] = true;
}
}
});
/* Blog section view tracking */
new Waypoint({
element: document.getElementById('blog'),
handler: function() {
if(!parallaxEventLog['blog']) {
ga('send', 'event', 'homepage', 'section_view', 'blog');
parallaxEventLog['blog'] = true;
}
}
});
/* Contact section view tracking */
new Waypoint({
element: document.getElementById('contact'),
handler: function() {
if(!parallaxEventLog['contact']) {
ga('send', 'event', 'homepage', 'section_view', 'contact');
parallaxEventLog['contact'] = true;
}
}
});
</script>
See the Event Tracking documentation for full details on the ga
tracking arguments.
Note The above is an over simplified example for demonstration purposes, it can be abstracted to reduce duplication
Viewing scroll events
- In the Google Analytics control panel go to Behaviour -> Events -> Overview then click view full report on the bottom left
3. Click homepage in the event category list
4. Click section_view in the event action list
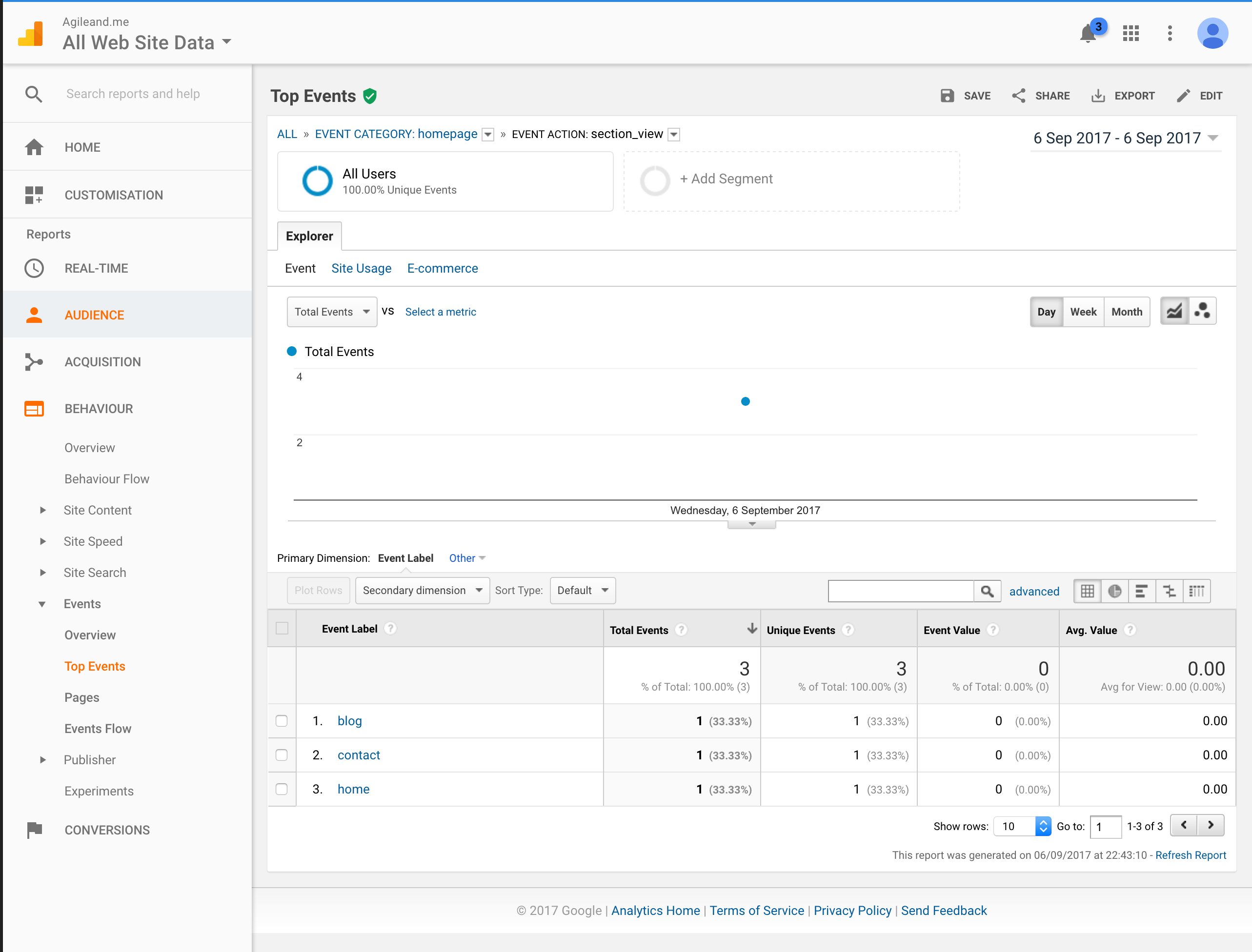
Tracking menu clicks
For tracking menu clicks the onclick property can be used.
Add onclick
properties to the menu.
<ul>
<li class="home"><a href="#home" onclick="menuClick('home')">Home</a></li>
<li class="blog"><a href="#blog" onclick="menuClick('blog')">Blog</a></li>
<li class="contact"><a href="#contact" onclick="menuClick('contact')">contact</a></li>
</ul>
Add the below script to the bottom of the page.
function menuClick(item){
ga('send', 'event', 'homepage', 'menu_click', item);
}